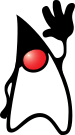
The Java programming course
Java is a programming language originally developed by James Gosling at Sun Microsystems and released in 1995. It is an object-oriented programming language. The language derives much of its syntax from C++ but has a simpler object model and fewer low-level facilities.
Prerequisites
Students should know:
- C++ programming language;
- basic data structures (arrays, lists, trees, graphs).
Contents of the course
- types, values, variables, operators, expressions, control flow
- program structure and packages
- strings and regular expressions
- classes and objects
- static members
- extending classes
- interfaces
- nested classes and interfaces
- exceptions and assertions
- AWT/Swing GUI components
- event handling
- designing with MVC
- applets
- enumeration types
- generic types
- collections
- streams and serialization
- threads
- reflection
- beans
References
Books:
- Ken Arnold, James Gosling, David Holmes: The Java programming language. Fourth edition. Prentice Hall PTR, 2005.
- Bruce Eckel: Thinking in Java. Fourth edition. Prentice Hall, 2006.
- James Gosling, Bill Joy, Guy Steele, Gilad Bracha: The Java Language Specification. Third Edition. McGraw-Hill, 2005.
- Tim Lindholm, Frank Yellin: The Java Virtual Machine Specification. Second Edition. Prentice Hall PTR, 1999.
- Herbert Schildt: Java 2: the complete reference. Fifth Edition. McGraw-Hill, 2002.
- Jonathan Knudsen, Patrick Niemeyer: Learning Java. Third Edition. O'Reilly, 2005.
- Sharon Zakhour, Scott Hommel, Jacob Royal, Isaac Rabinovitch, Tom Risser, Mark Hoeber: The Java Tutorial. Fourth Edition. Addison Wesley Professional, 2006.
- Ivor Horton: Ivor Horton's Beginning Java 2, JDK 5 edition. Wiley Publishing, 2005.
Basic references:
Further references:
Internet:
- Java Platform SE6 API Specifications (java.sun.com)
- Java SE Technologies at a Glance (java.sun.com)
- The Java Tutorials (java.sun.com)
Schedule
- Lecture: Monday 14-16, room 105
- Lab: Monday 16-18, room 107
Advertisements
- 15.01.2010
- Dear Students! You can get extra points programming the additional 10th exercise.
- 13.01.2010
- Dear Students! The 9th exercise, the last one, is available.
- 4.01.2010
- Dear Students! A new exercise, and the first in this year, is available.
- 13.11.2009
- Dear Students! Near Monday (16 November 2009) is a free day at our University and there are no lectures and no exercises in this day.
- 09.11.2009
- Dear Students! You should read the lecture notes and prepare programs week by week. Please, do it!
- 01.10.2009
- Here is the place where important news will be published.
Labs
Rules
- general:
- At this site several simple programming tasks will be published. For each properly programmed task the student can score up to 10 points or up to 5 points for the task submitted within a week after the deadline.
- deadline:
- The deadline for each task is one week after its publication. Delays longer than a week are not acceptable. The only exceptions are: illness confirmed by a doctor, official matters confirmed by the appropirate authorities.
- presentation of solutions:
- Programs have to be presented during lab sessions, personally by the author (student). It is unacceptable to pass the solutions by colleagues. During the presentation the author should be prepared to answer questions about: the method, specific langauge constructions, technologies, etc. used in the proposed solution.
- programs:
- Presented programs have to be sent to me immediately after the lab sessions. I will collect your programs to the end of semester.
- evaluation:
- To receive the credit for this course, grade 3 (sufficient), the student has to collect minimum 50% of all the possible points; for grade 5 (very good) it is necessary to collect 90% of all the possible points; the rest of the intermediate grades are linearly dependent on the above border requirements.
Exercises
-
5.10.2009: no task
- 12.10.2009: a number to words converter (ps/pdf)
- 19.10.2009: polynomials (ps/pdf)
- 26.10.2009: Pascal's triangle (ps/pdf) 2.11.2009: no task
- 9.11.2009: expression trees (ps/pdf)
- 23.11.2009: graph representations (ps/pdf) 30.11.2009: no task
- 7.12.2009: an integer calculator (ps/pdf) 14.12.2009: no task
- 21.12.2009: a coloured drawing (ps/pdf)
- 11.01.2009: a maze (ps/pdf)
- 18.01.2009: a file navigator (ps/pdf)
- 25.01.2009: Bastille Solitaire (ps/pdf)
Ranking
- the english group of "Java": (html)
Lectures
- 5.10.2009 Java progamming language:
-
- overview of Java
- the standard library
- standard input/output
- writing data to output stream
- reading data from input stream
- the String class
- arrays
- 12/19.10.2009 classes and objects:
-
- class definition
- class members: fields and methods
- access control
- creating objects
- garbage-collector
- construction and initialization
- the this reference
- methods and their arguments
- static members
- overloading methods
- the main method
- 26.10/2.11.2009 extending classes:
-
- extended classes
- constructors in extended classes
- inheriting and redefining members: overriding methods, hiding fields
- type compatibility and conversion
- testing for type using instanceof operator
- marking methods and classes final
- abstract classes and methods
- the Object class
- cloning objects
- 2.11.2009 interfaces:
-
- interfaces as an expression of pure design
- interface declarations
- extending interfaces
- working with interfaces
- marker interfaces
- 9.11.2009 nested classes and interfaces:
-
- static nested types (classes and interfaces)
- inner classes
- accessing enclosing objects
- extending inner classes
- local inner classes
- anonymous inner classes
- 23.11.2009 exceptions and assertions:
-
- exception types
- the throw statement
- the throws clause
- the tty-catch-finally statement
- the assert statement
- turning assertions on and off
- 30.11.2009 AWT:
-
- AWT - the original user interface toolkit
- components and containers in the AWT package
- layout managers
- simple window applications (Frame and Dialog objects)
- events
- 7.12.2009 Swing:
-
- Swing - the advanced user interface toolkit
- various components in the Swing package
- top-level containers in the Swing package
- painting in JPanel
- creating and showing simple dialogs using JOptionPane
- menus
- modifying the look and feel
- 14/21.12.2009 applets:
-
- defining an applet subclass
- life cycle of an applet
- an applet runs in the context of a browser
- defining and using applet parameters
- what applets can and cannot do
- finding and loading data files
- displaying short status strings
- displaying documents in the browser
- 4/11.01.2010 streams:
-
- streams overview
- byte streams
- character streams
- the conversion streams InputStreamReader and OutputStreamWriter
- filter streams
- the data byte streams (DataInput and DataOutput)
- the IOException classes
- working with files
- object serialization (the Serializable interface)
- 17.01.2010 generics and collection framework:
-
- generic types
- the Collection interface
- the Map interface
- iterators
- the for-each loop
- object ordering
- collection implementations
- algorithms
- 25.01.2010 threads:
-
- multithreading
- creating threads
- life cycle of an applet
- synchronization
- wait, notifyAll, and notify methods
- thread scheduling
- deadlocks
- ending thread execution
- synchronization and volatile
- thread management